Integrate the Mintlify widget into your products to offer users quick access to AI-powered chat with your docs content as the knowledge base.
Getting started
First, generate an API key in the Mintlify dashboard.
Installation
Add the widget by adding these script tags into your site’s <head>...<head/>
tag.
<script>
window.mintlifyWidgetSettings = {
connection: {
apiKey: 'Your API key here'
},
};
</script>
<script>
(function () {
if (!document.getElementById('mintlify-widget')) {
var script = document.createElement('script');
script.id = 'mintlify-widget';
script.src = 'https://unpkg.com/@mintlify/widget@^0';
script.onload = () => MintlifyWidget.init();
document.head.appendChild(script);
}
})();
</script>
To use the widget in React and Next.js apps, use the React component from the @mintlify/widget-react
package. Here is a basic example of how to use the component in your React application:
<MintlifyWidget
connection={{
apiKey: 'Your API key here',
}}
/>
Usage
In the first script tag or the React component props, you can customize the appearance and other settings of the widget. mintlifyWidgetSettings
accepts the following props:
Prop | Type | Description |
---|
apiKey | string | Widget API key generated from Mintlify dashboard. Required. |
url? | string | Used for internal testing only |
Prop | Type | Description |
---|
type? | 'button' |'input' | Type of the trigger to display. Defaults to button . |
label? | string | Label displayed in the trigger. Defaults to Get help for the button trigger and Ask anything... for the input trigger. |
buttonIcon? | 'chat' |'sparkles' |'mintlify' | Icon used in the trigger. Only available for the button trigger. Defaults to chat . |
iconOnly? | boolean | Only show icon in the trigger or not. Defaults to false . |
Here is an overview of what the trigger looks like with different configurations.
type='input' | |
---|
|  |
type='button' | 'chat' | 'sparkles' | 'mintlify' |
---|
iconOnly=false |  | 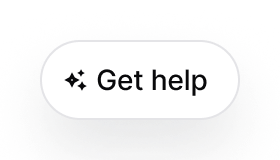 | 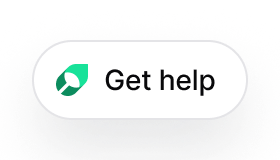 |
iconOnly=true |  | 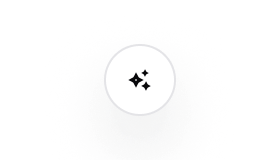 | 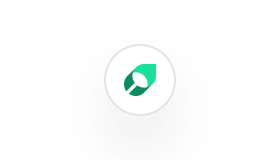 |
Prop | Type | Description |
---|
primary? | string | Primary color used in the widget. Defaults to #0D9373 . |
primaryLight? | string | Primary color in dark mode. Defaults to #55D799 . |
Prop | Type | Description |
---|
openCitationInSameTab? | boolean | Open the citation url in the same tab or not. Defaults to false . |
exampleQueries? | string[] | Example queries to prompt the user to ask. Defaults to [] . |
Prop | Type | Description |
---|
trackChatEnter | ()=> void | Triggered when the user opens the chat widget. |
trackCitationClick | (title: string, url: string)=> void | Triggered when the user clicks on a citation. |
trackChatThumbsUp | (query: string, response: string)=> void | Triggered when the user thumbs up on a response. |
trackChatThumbsDown | (query: string, response: string)=> void | Triggered when the user thumbs down on a response. |
trackChatFollowup | (query: string)=> void | Triggered when the user asks a question. |
trackChatClose | (queriesCount: number)=> void | Triggered when the user exits the chat widget. |